Do you know that website like The White House , Disney, Facebook Newsroom, MTV and some many more popular website use WordPress Content management system (CMS) to power their websites?
Yes! WordPress is an awesome CMS.
However, the core CMS does provide a lot of functional flexibility, the core software still lacks a certain degree of functionality.
The core software of WordPress is a bit like an incomplete puzzle. WordPress plugins are the missing part.
But the biggest advantage is a lot of free and paid plugins are readily available to use and you just have to install and use them.
Plugins are like add-ons or bit of codes which can be installed to a WordPress. Users can install them to improve their WordPress site in various ways, including article enhancement, security, managing comments, and optimizing content to rank higher in search engines.
In short, it can make a website look more professional, run faster, and enhance the features.
The good news is there are more than 55,000 plugins to choose from the WordPress plugin directory and there are at least a thousand on third-party websites.
The bad news is how do you choose the best WordPress plugins to use?
Depending on your business you may require different plugins which different features and functionality.This guide brings you the best seven plugins that we believe every WordPress user should have.
List of Top 7 Best WordPress Plugins
- WP Rocket – The Best for Speed Optimization
- Rank Math – The Best for Increasing Organic Website Traffic
WP Rocket
Speed Optimization is one of the important factors in SEO from the aspect of Technical SEO. WP Rocket is a plugin, used by millions of websites and helpful for making your WordPress good at speed.
Most of the WordPress users are not aware of the coding for making their website optimized. For example, Removal of unnecessary code, etc.
This plugin will automatically help you in speeding up your loading speed by making the necessary actions. Here are the features of this plugin:
- It removes the cache of the page
- It preloads Sitemap
- It compresses the GZIP
- It optimizes the database for faster fetching
- Minify of CSS, JS loading
- CDN integration
- DNS Prefetching
There are a lot of other features of this plugin that will force you to buy the premium account of this plugin.
Rank Math
Rank Math is one of the most effective SEO plugins for WordPress users. It allows you to manage the On-Page SEO of your website.
The biggest advantage of this plugin is that it gets integrated with Google Search Console and you can get some essential information directly on your dashboard.

It helps to manage the tags like no-index, no-follow, no-archive. The plugin would also help you to focus on the specific keyword.
Some of the features of Rank Math includes XML sitemap, Meta Description, Links, 404 errors, Redirects, Local SEO, Image SEO.
Prepostseo
We all know that the SEO factors are usually considered on the content and if your content is not potential then your SEO wouldn’t be so effective.
Before applying the SEO implications, you should consider making your content up to the mark and Prepostseo can help you in this regard.

Here are some of the top features of Prepostseo:
- It checks your content in the sense of SEO like readability score and some other optimization score
- It checks the plagiarism in your content and checks the ratio of the plagiarism and unique content in your writing
- It helps to remove the grammatical errors in your writing through providing the suggestion upon mistakes
- It finds the broken links in your web pages which is an important SEO application
- Besides these, there are a lot of other features for your website like counting the words and character of your content.
SEMrush SEO Writing Assistant
SEMrush is one of the popular brands for SEO experts and webmasters. They are also offering the WordPress plugin for WordPress users and through this, you can improve your writing skills.
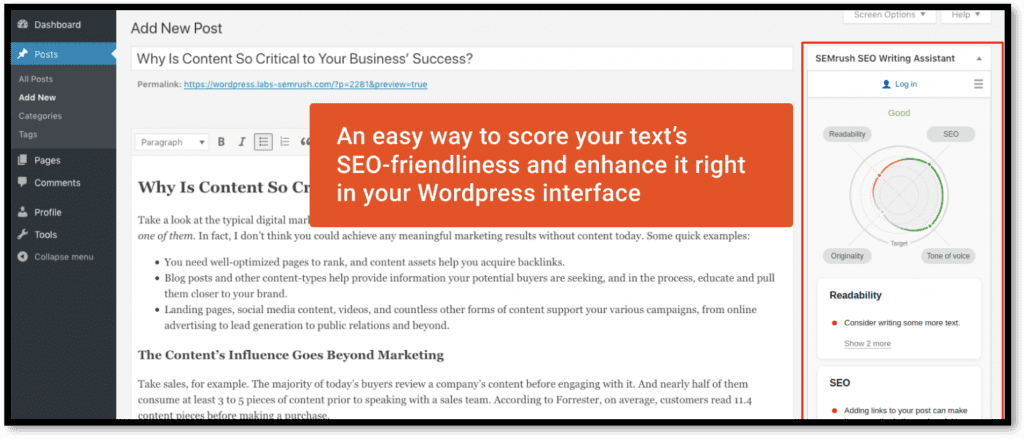
This plugin helps make your content up to the Search engine algorithms. Some of the features of this amazing plugin are discussed below:
- Firstly, it will analyze your content and give you a score for that content according to the readability and SEO measurement.
- It suggests making your content friendly to read which is helpful for the audience and crawlers to understand.
- For better SEO, it will also help you to make some changes in your content for better optimization.
- It will allow you to add the keyword and get suggestions for making your content accordingly.
- The biggest advantage of this amazing plugin is that it will let you know how much word count for the article you should have for the specific topic. Moreover, it will also let you know the number of keywords you should have in the content.
Broken Link checker
Broken links are one of the bad factors for SEO and users. Broken links are the external links that are placed in your content but the navigated web page though the link is not available.
For example, you are placing the link to another site but the other web page or post is removed. These broken links are harmful to the ranking of your website because crawlers are very familiar with the links.
This plugin will provide the feature of monitoring the broken links whether it’s pointing to the web page, comment, or any post.
In case you are having a broken link, this plugin will stop the crawlers to follow the broken link for affecting your SEO.
Conclusion
As we know that WordPress is famous because of the access to plugins and readymade themes. On other platforms or through the programming language, it is quite tough to have the SEO factors applied to your site.
However, the case is completely different on WordPress because the majority of the process is done through the plugins.
The above-mentioned plugins can help you to make your website optimize to the search engine for better ranking.
Most of the plugins which are discussed in this article are free to use while you can go for the premium if you are loving these plugins.